to store multiple values in same custom field key and print them at
once
If you want to store it as site option, you can use update_option()
:
http://codex.wordpress.org/Function_Reference/update_option
Example 1:
// some array to store:
$items=array('yellow','orange','green');
// save the array
update_option('myitems',$items);
// get the array
$items=get_option('myitems');
// print the array
echo "<ul>";
foreach($items as $item){
echo "<li>".$item."</li>";
}
echo "</ul>";
If you want to store it as post meta (i.e. for each post), you can use update_post_meta()
http://codex.wordpress.org/Function_Reference/update_post_meta
Example 2:
// some array to store:
$items=array('yellow','orange','green');
// save the array
update_post_meta($post_id,'myitems',$items);
// get the array
$items = get_post_meta($post_id,'myitems',true);
// print the array
echo "<ul>";
foreach($items as $item){
echo "<li>".$item."</li>";
}
echo "</ul>";
Example 3:
If you want to add the custom fields (same meta key) and values from the backend like this:
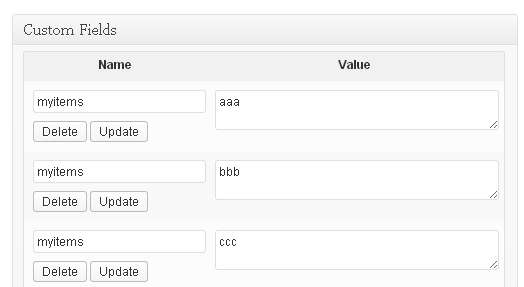
you can retreieve the values like this:
// get the array for current post
$items = get_post_meta($post->ID,'myitems'); // we skip the true part here
// print the array
echo "<ul>";
foreach($items as $item){
echo "<li>".$item."</li>";
}
echo "</ul>";